Django: uniform image resizing
Sometimes, you want all the thumbnails you're creating to be the same dimensions. For example, your layout may require a certain width so that all the items line up in a grid. In my case, I wanted them to be exactly 96x96 pixels.
The tool of choice in Django is PIL, which is a PITA to install, but which works well once it's installed. Just be sure to check that JPEG works, it's commonly flubbed by the installer.
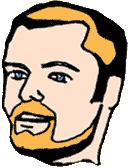
Another common requirement is to retain the aspect ratio of the original image. For example, given the above input image, we would not want to produce this:

For that, PIL's built-in thumbnail method will do nicely. However, that would not get us a perfect 96x96 square. Unless the original image is also a square, it will instead produce a thumbnail where only the larger dimension it 96 pixels.
To compensate, we need to add literal white space to fill out the remaining pixels. In PIL, you can accomplish this with the paste method.
import Image import traceback import sys def resize_aspect_ratio(path, maxSize, method=Image.ANTIALIAS): """ Resizing an image to a static side, in place """ try: # shrink, but maintain aspect ratio image = Image.open(path) image.thumbnail(maxSize) # fill out to exactly maxSize (square image) background = Image.new("RGBA", maxSize, (255, 255, 255, 0)) # use white for empty space background.paste(image, ((maxSize[0] - image.size[0]) / 2, (maxSize[1] - image.size[1]) / 2)) background.save(path) except Exception, e: print "Error resizing image: %s" % e
